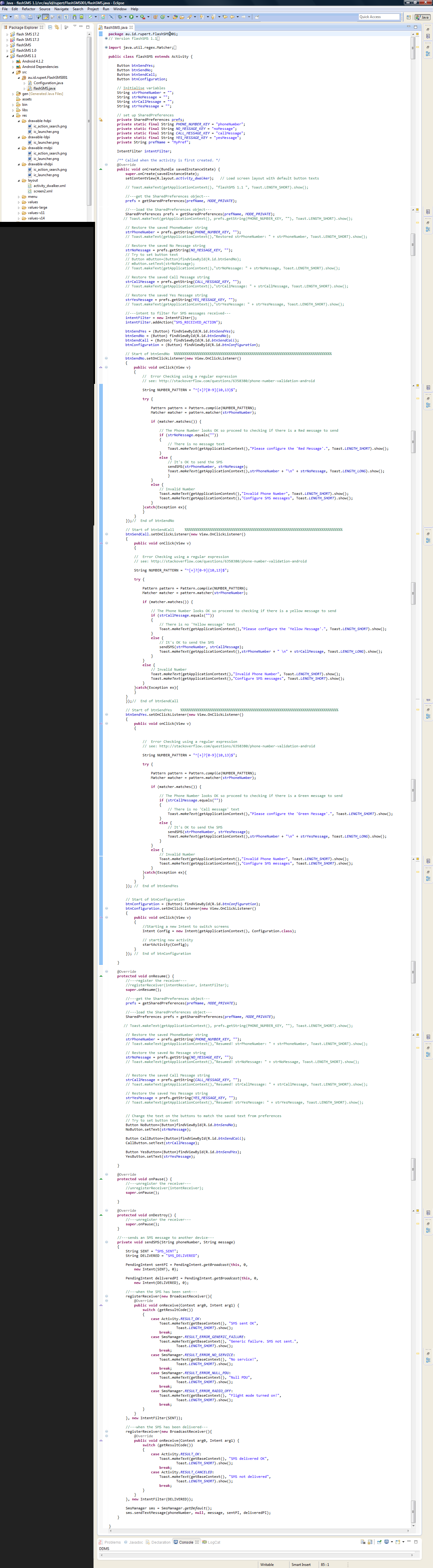 |
package au.id.rupert.FlashSMS001;
// Version flashSMS 1.1
// Purpose to send one of 3 predefined SMS messages to a predefined phone number
// This is the version I am publishing 30 Dec 2012 17:30
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import android.app.Activity;
import android.app.PendingIntent;
import android.content.BroadcastReceiver;
import android.content.Context;
import android.content.Intent;
import android.content.IntentFilter;
import android.content.SharedPreferences;
import android.os.Bundle;
import android.telephony.SmsManager;
import android.view.View;
import android.widget.Button;
import android.widget.Toast;
public class flashSMS extends Activity {
Button btnSendYes;
Button btnSendNo;
Button btnSendCall;
Button btnConfiguration;
// Initialise variables
String strPhoneNumber = "";
String strNoMessage = "";
String strCallMessage = "";
String strYesMessage = "";
// set up SharedPreferences
private SharedPreferences prefs;
private static final String PHONE_NUMBER_KEY = "phoneNumber";
private static final String NO_MESSAGE_KEY = "noMessage";
private static final String CALL_MESSAGE_KEY = "callMessage";
private static final String YES_MESSAGE_KEY = "yesMessage";
private String prefName = "MyPref";
IntentFilter intentFilter;
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_dwalker); // Load screen layout with default button texts
// Toast.makeText(getApplicationContext(), "flashSMS 1.1 ", Toast.LENGTH_SHORT).show();
//---get the SharedPreferences object---
prefs = getSharedPreferences(prefName, MODE_PRIVATE);
//---load the SharedPreferences object---
SharedPreferences prefs = getSharedPreferences(prefName, MODE_PRIVATE);
// Toast.makeText(getApplicationContext(), prefs.getString(PHONE_NUMBER_KEY, ""), Toast.LENGTH_SHORT).show();
// Restore the saved PhoneNumber string
strPhoneNumber = prefs.getString(PHONE_NUMBER_KEY, "");
// Toast.makeText(getApplicationContext(),"Restored strPhoneNumber: " + strPhoneNumber, Toast.LENGTH_SHORT).show();
// Restore the saved No Message string
strNoMessage = prefs.getString(NO_MESSAGE_KEY, "");
// Try to set button text
// Button mButton=(Button)findViewById(R.id.btnSendNo);
// mButton.setText(strNoMessage);
// Toast.makeText(getApplicationContext(),"strNoMessage: " + strNoMessage, Toast.LENGTH_SHORT).show();
// Restore the saved Call Message string
strCallMessage = prefs.getString(CALL_MESSAGE_KEY, "");
// Toast.makeText(getApplicationContext(),"strCallMessage: " + strCallMessage, Toast.LENGTH_SHORT).show();
// Restore the saved Yes Message string
strYesMessage = prefs.getString(YES_MESSAGE_KEY, "");
// Toast.makeText(getApplicationContext(),"strYesMessage: " + strYesMessage, Toast.LENGTH_SHORT).show();
//---intent to filter for SMS messages received---
intentFilter = new IntentFilter();
intentFilter.addAction("SMS_RECEIVED_ACTION");
btnSendYes = (Button) findViewById(R.id.btnSendYes);
btnSendNo = (Button) findViewById(R.id.btnSendNo);
btnSendCall = (Button) findViewById(R.id.btnSendCall);
btnConfiguration = (Button) findViewById(R.id.btnConfiguration);
// Start of btnSendNo %%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
btnSendNo.setOnClickListener(new View.OnClickListener()
{
public void onClick(View v)
{
// Error Checking using a regular expression
// see: http://stackoverflow.com/questions/6358380/phone-number-validation-android
String NUMBER_PATTERN = "^[+]?[0-9]{10,13}$";
try {
Pattern pattern = Pattern.compile(NUMBER_PATTERN);
Matcher matcher = pattern.matcher(strPhoneNumber);
if (matcher.matches()) {
// The Phone Number looks OK so proceed to checking if there is a Red message to send
if (strNoMessage.equals(""))
{
// There is no message text
Toast.makeText(getApplicationContext(),"Please configure the 'Red Message'.", Toast.LENGTH_SHORT).show();
}
else {
// It's OK to send the SMS
sendSMS(strPhoneNumber, strNoMessage);
Toast.makeText(getApplicationContext(),strPhoneNumber + "\n" + strNoMessage, Toast.LENGTH_LONG).show();
}
}
else {
// Invalid Number
Toast.makeText(getApplicationContext(),"Invalid Phone Number", Toast.LENGTH_SHORT).show();
Toast.makeText(getApplicationContext(),"Configure SMS messages", Toast.LENGTH_SHORT).show();
}
}catch(Exception ex){
}
}
});// End of btnSendNo
// Start of btnSendCall %%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
btnSendCall.setOnClickListener(new View.OnClickListener()
{
public void onClick(View v)
{
// Error Checking using a regular expression
// see: http://stackoverflow.com/questions/6358380/phone-number-validation-android
String NUMBER_PATTERN = "^[+]?[0-9]{10,13}$";
try {
Pattern pattern = Pattern.compile(NUMBER_PATTERN);
Matcher matcher = pattern.matcher(strPhoneNumber);
if (matcher.matches()) {
// The Phone Number looks OK so proceed to checking if there is a yellow message to send
if (strCallMessage.equals(""))
{
// There is no 'Yellow message' text
Toast.makeText(getApplicationContext(),"Please configure the 'Yellow Message'.", Toast.LENGTH_SHORT).show();
}
else {
// It's OK to send the SMS
sendSMS(strPhoneNumber, strCallMessage);
Toast.makeText(getApplicationContext(),strPhoneNumber + " \n" + strCallMessage, Toast.LENGTH_LONG).show();
}
}
else {
// Invalid Number
Toast.makeText(getApplicationContext(),"Invalid Phone Number", Toast.LENGTH_SHORT).show();
Toast.makeText(getApplicationContext(),"Configure SMS messages", Toast.LENGTH_SHORT).show();
}
}catch(Exception ex){
}
}
});// End of btnSendCall
// Start of btnSendYes %%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
btnSendYes.setOnClickListener(new View.OnClickListener()
{
public void onClick(View v)
{
// Error Checking using a regular expression
// see: http://stackoverflow.com/questions/6358380/phone-number-validation-android
String NUMBER_PATTERN = "^[+]?[0-9]{10,13}$";
try {
Pattern pattern = Pattern.compile(NUMBER_PATTERN);
Matcher matcher = pattern.matcher(strPhoneNumber);
if (matcher.matches()) {
// The Phone Number looks OK so proceed to checking if there is a Green message to send
if (strCallMessage.equals(""))
{
// There is no 'Call message' text
Toast.makeText(getApplicationContext(),"Please configure the 'Green Message'.", Toast.LENGTH_SHORT).show();
}
else {
// It's OK to send the SMS
sendSMS(strPhoneNumber, strYesMessage);
Toast.makeText(getApplicationContext(),strPhoneNumber + "\n" + strYesMessage, Toast.LENGTH_LONG).show();
}
}
else {
// Invalid Number
Toast.makeText(getApplicationContext(),"Invalid Phone Number", Toast.LENGTH_SHORT).show();
Toast.makeText(getApplicationContext(),"Configure SMS messages", Toast.LENGTH_SHORT).show();
}
}catch(Exception ex){
}
}
}); // End of btnSendYes
// Start of btnConfiguration
btnConfiguration = (Button) findViewById(R.id.btnConfiguration);
btnConfiguration.setOnClickListener(new View.OnClickListener()
{
public void onClick(View v)
{
//Starting a new Intent to switch screens
Intent Config = new Intent(getApplicationContext(), Configuration.class);
// starting new activity
startActivity(Config);
}
}); // End of btnConfiguration
}
@Override
protected void onResume() {
//---register the receiver---
//registerReceiver(intentReceiver, intentFilter);
super.onResume();
//---get the SharedPreferences object---
prefs = getSharedPreferences(prefName, MODE_PRIVATE);
//---load the SharedPreferences object---
SharedPreferences prefs = getSharedPreferences(prefName, MODE_PRIVATE);
// Toast.makeText(getApplicationContext(), prefs.getString(PHONE_NUMBER_KEY, ""), Toast.LENGTH_SHORT).show();
// Restore the saved PhoneNumber string
strPhoneNumber = prefs.getString(PHONE_NUMBER_KEY, "");
// Toast.makeText(getApplicationContext(),"Resumed! strPhoneNumber: " + strPhoneNumber, Toast.LENGTH_SHORT).show();
// Restore the saved No Message string
strNoMessage = prefs.getString(NO_MESSAGE_KEY, "");
// Toast.makeText(getApplicationContext(),"Resumed! strNoMessage: " + strNoMessage, Toast.LENGTH_SHORT).show();
// Restore the saved Call Message string
strCallMessage = prefs.getString(CALL_MESSAGE_KEY, "");
// Toast.makeText(getApplicationContext(),"Resumed! strCallMessage: " + strCallMessage, Toast.LENGTH_SHORT).show();
// Restore the saved Yes Message string
strYesMessage = prefs.getString(YES_MESSAGE_KEY, "");
// Toast.makeText(getApplicationContext(),"Resumed! strYesMessage: " + strYesMessage, Toast.LENGTH_SHORT).show();
// Change the text on the buttons to match the saved text from preferences
// Try to set button text
Button NoButton=(Button)findViewById(R.id.btnSendNo);
NoButton.setText(strNoMessage);
Button CallButton=(Button)findViewById(R.id.btnSendCall);
CallButton.setText(strCallMessage);
Button YesButton=(Button)findViewById(R.id.btnSendYes);
YesButton.setText(strYesMessage);
}
@Override
protected void onPause() {
//---unregister the receiver---
//unregisterReceiver(intentReceiver);
super.onPause();
}
@Override
protected void onDestroy() {
//---unregister the receiver---
super.onPause();
}
//---sends an SMS message to another device---
private void sendSMS(String phoneNumber, String message)
{
String SENT = "SMS_SENT";
String DELIVERED = "SMS_DELIVERED";
PendingIntent sentPI = PendingIntent.getBroadcast(this, 0,
new Intent(SENT), 0);
PendingIntent deliveredPI = PendingIntent.getBroadcast(this, 0,
new Intent(DELIVERED), 0);
//---when the SMS has been sent---
registerReceiver(new BroadcastReceiver(){
@Override
public void onReceive(Context arg0, Intent arg1) {
switch (getResultCode())
{
case Activity.RESULT_OK:
Toast.makeText(getBaseContext(), "SMS sent OK",
Toast.LENGTH_SHORT).show();
break;
case SmsManager.RESULT_ERROR_GENERIC_FAILURE:
Toast.makeText(getBaseContext(), "Generic failure. SMS not sent.",
Toast.LENGTH_SHORT).show();
break;
case SmsManager.RESULT_ERROR_NO_SERVICE:
Toast.makeText(getBaseContext(), "No service!",
Toast.LENGTH_SHORT).show();
break;
case SmsManager.RESULT_ERROR_NULL_PDU:
Toast.makeText(getBaseContext(), "Null PDU",
Toast.LENGTH_SHORT).show();
break;
case SmsManager.RESULT_ERROR_RADIO_OFF:
Toast.makeText(getBaseContext(), "Flight mode turned on!",
Toast.LENGTH_SHORT).show();
break;
}
}
}, new IntentFilter(SENT));
//---when the SMS has been delivered---
registerReceiver(new BroadcastReceiver(){
@Override
public void onReceive(Context arg0, Intent arg1) {
switch (getResultCode())
{
case Activity.RESULT_OK:
Toast.makeText(getBaseContext(), "SMS delivered OK",
Toast.LENGTH_SHORT).show();
break;
case Activity.RESULT_CANCELED:
Toast.makeText(getBaseContext(), "SMS not delivered",
Toast.LENGTH_SHORT).show();
break;
}
}
}, new IntentFilter(DELIVERED));
SmsManager sms = SmsManager.getDefault();
sms.sendTextMessage(phoneNumber, null, message, sentPI, deliveredPI);
}
} |